Vanna: Generating Accurate SQL Queries from Text Using RAG Techniques
General Introduction
Vanna is an MIT-licensed open source Python framework focused on generating SQL queries through RAG (Retrieval Augmented Generation) techniques. Users can train RAG models, apply them to their own data, and then ask questions, and Vanna will return corresponding SQL queries. These queries can be automatically run in the database, simplifying complex database operations.Vanna supports a wide range of Large Language Models (LLMs) and vector storage solutions for a variety of databases, such as PostgreSQL, MySQL, Snowflake, and others.
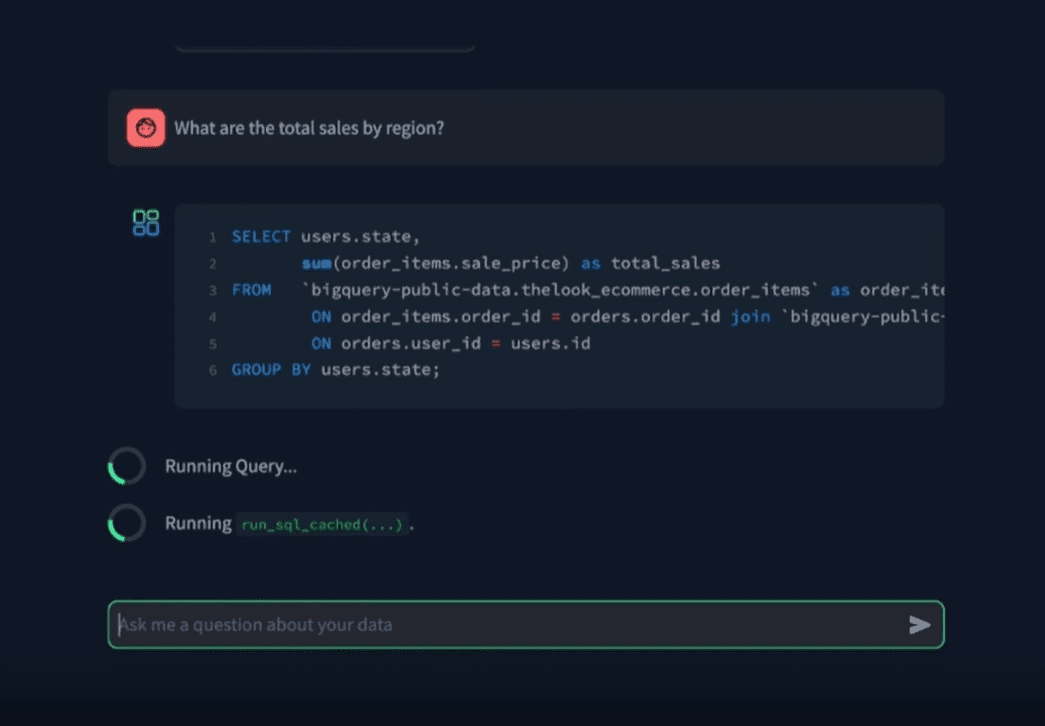
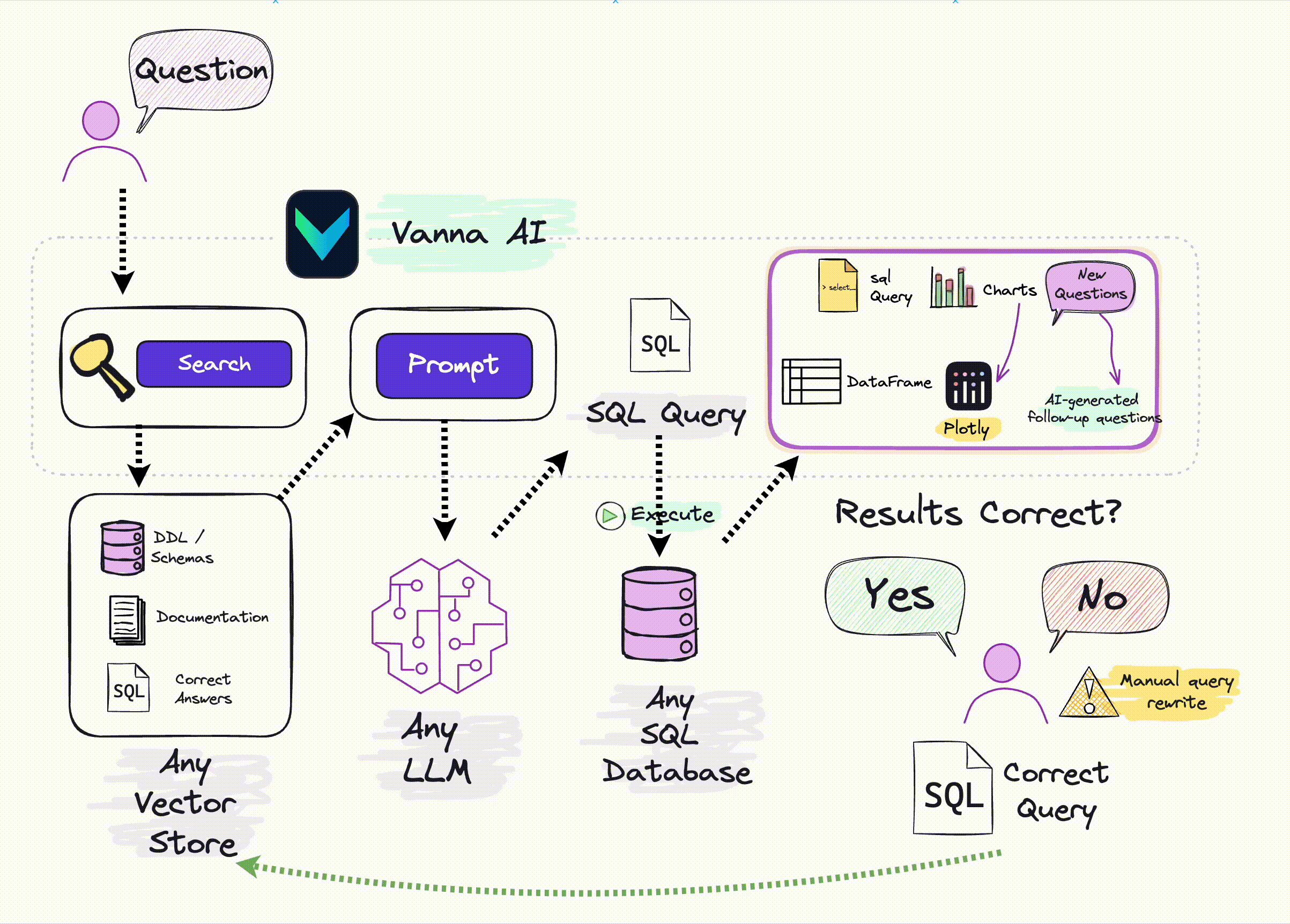
Function List
- SQL Generation: Generate precise SQL queries through natural language.
- RAG model training: Users can train their own RAG models to fit specific data.
- Multi-LLM Support: Compatible with OpenAI, Anthropic, HuggingFace and many other LLMs.
- Multi-vector storage support: Support for AzureSearch, PineCone, ChromaDB, and other vector stores.
- Multi-database support: Compatible with PostgreSQL, MySQL, Snowflake and many other databases.
- user: Provides Jupyter Notebook, Streamlit, Flask and other user interfaces.
Using Help
Installation process
- Installation of Vanna: Install Vanna using pip.
pip install vanna
- Installation of optional packages: Install other optional packages as needed, please refer to the documentation for details.
Usage Process
- Import Vanna: Import the appropriate modules according to the LLM and vector database used.
from vanna.openai_chat import OpenAI_Chat
from vanna.chromadb import ChromaDB_VectorStore
class MyVanna(ChromaDB_VectorStore, OpenAI_Chat):
def __init__(self, config=None):
ChromaDB_VectorStore.__init__(self, config=config)
OpenAI_Chat.__init__(self, config=config)
vn = MyVanna(config={'api_key': 'sk-...', 'model': 'gpt-4-...'})
- Training the RAG model: Run training commands as needed.
vn.train(ddl_statements)
- Generating SQL Queries: Ask a question and Vanna will return the appropriate SQL query.
sql_query = vn.ask("给我所有客户的名字")
Detailed Function Operation
- SQL GenerationVanna is a natural language application that allows users to type in questions in natural language and Vanna will automatically generate SQL queries for them. For example, type in "Give me the names of all my customers" and Vanna will generate the corresponding SQL query.
- RAG model training: Users can train RAG models based on their data to improve the accuracy of SQL generation. The training process involves providing DDL statements based on which Vanna will understand the structure and relationships of the database.
- Multi-LLM Support: Vanna is compatible with a wide range of large language models, allowing users to select the appropriate model for SQL generation as needed.
- Multi-vector storage support: Vanna supports a wide range of vector storage solutions.
- Multi-database support: Vanna is compatible with a wide range of databases and can be configured and used by users according to their database type.
- user: Vanna provides a variety of user interfaces, users can choose Jupyter Notebook, Streamlit, Flask and other interfaces to operate conveniently.
sample code (computing)
Below is a complete sample code showing how to generate SQL queries using Vanna:
from vanna.openai_chat import OpenAI_Chat
from vanna.chromadb import ChromaDB_VectorStore
class MyVanna(ChromaDB_VectorStore, OpenAI_Chat):
def __init__(self, config=None):
ChromaDB_VectorStore.__init__(self, config=config)
OpenAI_Chat.__init__(self, config=config)
vn = MyVanna(config={'api_key': 'sk-...', 'model': 'gpt-4-...'})
# 训练RAG模型
vn.train(ddl_statements)
# 生成SQL查询
sql_query = vn.ask("给我所有客户的名字")
print(sql_query)
What is Function RAG in SQL generation?
Vanna is pleased to introduce a breakthrough feature in SQL generation: Function RAG (Search Enhanced Generation). This new optional experimental feature is designed to enhance the consistency and determinism of SQL generation to meet the many requests from our users.
What is Function RAG?
Function RAG converts traditional Question-SQL training pairs into callable templates (aka functions/tools). The Large Language Model (LLM) then calls these templates to generate SQL queries and any associated post-processing code (e.g., charting code). Crucially, when using the Function RAG, the Large Language Model (LLM) only determines the SQL templates to be used and any parameters that need to be provided. This approach not only ensures more consistent output, but also significantly speeds up the SQL generation process.
We are releasing the Function RAG API under a different domain and brand to distinguish it from the core open source Python packages.
Key Features of Function RAG
- Template-based SQL generation: By converting training pairs into templates, Function RAG ensures that the generated SQL is both accurate and relevant to the user's query.
- Enhanced Security: Function RAG reduces hint injection and hint escape, ensuring that the SQL generation process is protected from external manipulation.
- User-specific queries: Users can now pass information such as user IDs in queries without the risk of this data being overwritten. This allows personalized questions such as "What are my last 10 orders?" to be executed securely and efficiently. .
- Integrated Chart Code Generation: In addition to SQL, Function RAG handles the generation of charting code so that SQL and its corresponding visualization code can be generated in a single request.
- Multi-language support: This functionality is accessible through the GraphQL API, making it possible to use it not only from Python, but from any programming language. This opens up the possibility of integration into various backends, including frameworks such as Ruby on Rails, .
practical application
For example, here are some sample functions:
When you ask a question.vn.get_function(question=...)<span> </span>
The most suitable function will be found and the necessary parameters will be filled in using the Large Language Model (LLM).
To limit the issue to a specific user, you can use the vn.get_function(question=..., additional_data={"user_id": ...})<span> </span>
methodology. This will ensure that the user_id<span> </span>
parameter is set deterministically.
To create a new function, if you are doing it manually, you can use the vn.create_function(...)<span> </span>
method, or you can use the built-in web application to perform this action.
Manually created
vn.create_function(question=..., sql=..., plotly_code=...)
Web Application Function Creation
The web application has a new button that allows you to choose whether to train the results as raw Question-SQL pairs or as functions.The Function RAG will automatically extract what looks like a parameter from the question and make it available as a function parameter.
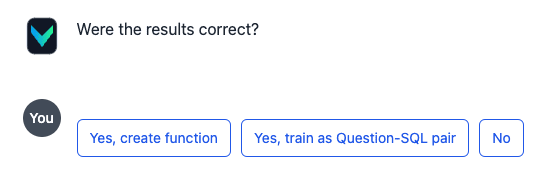
When to use
If you have variants where end users are asking similar questions and you only want users to be able to run specific types of analyses that have been manually approved by your engineering team, then the Function RAG is a great way to ensure that users can only run approved analyses.
Restricting to approved analytics helps end users feel confident that they are seeing the right data and viewing it in the right way. If you integrate it into a SaaS application, this allows you to put it in the hands of internal business users or end users.
Any user can benefit from the speed improvements offered by Function RAG. However, for data analysts, you may wish to fall back to the rest of Vanna's SQL generation functionality when performing novel analyses that are not present in large amounts of training data. If you are using a vector database hosted by Vanna, the fallback function is automatically included in the built-in web application with the parameter function_generation=True<span> </span>
The
GraphQL API Integration
In addition to the Python package, the Function RAG can be called using the GraphQL API, which makes it possible to use it in other languages and frameworks. The most common request we receive is the ability to use it in Ruby on Rails.
usability
Function RAG is available as part of Vanna's Free, Premium, and Enterprise Edition programs. You can find the Function RAG in the Vanna v0.6.0<span> </span>
and later versions to access it.
© Copyright notes
Article copyright AI Sharing Circle All, please do not reproduce without permission.
Related posts
No comments...