AI Chatbot: Vercel Builds and Deploys Intelligent Chatbots
General Introduction
AI Chatbot is an open source project developed by Vercel to help developers quickly build and deploy intelligent chatbots. The project is based on the Next.js framework and integrates a variety of AI model providers such as OpenAI, Anthropic and Cohere. by using Vercel's AI SDK, developers can easily generate text, structured objects and tool calls. The project also supports data persistence, file storage, and secure authentication, providing a complete solution for creating efficient, scalable chatbot applications.
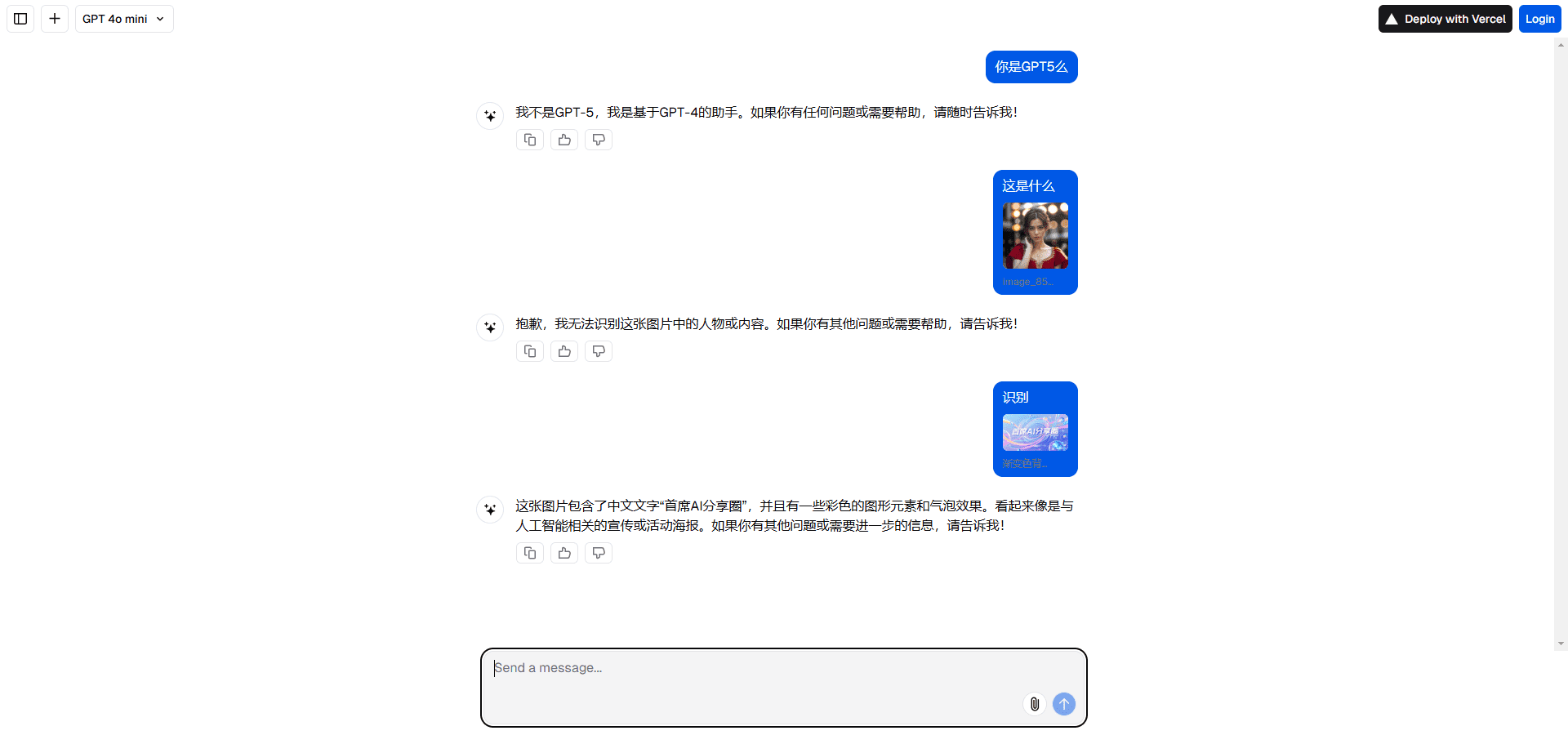
Function List
- Next.js Application Routing: Provides advanced routing capabilities for seamless navigation and high performance.
- React Server Components: Supports server-side rendering and server operations to improve performance.
- AI SDK: Unified API for generating text, structured objects and tool calls.
- Multi-model support: OpenAI GPT-4 is supported by default and can be switched to other model providers.
- Data persistence: Use Vercel Postgres to save chat logs and user data.
- File Storage: Efficient file storage with Vercel Blob.
- safety certification: Integrates with NextAuth.js to provide a simple and secure authentication mechanism.
- Styling: Provides flexible component styling using Tailwind CSS and Radix UI.
Using Help
Installation process
- Installing the Vercel CLI: Run in a terminal
npm i -g vercel
Install the Vercel command line tool. - Linking Local Instances: Use
vercel link
Link the local instance with Vercel and GitHub accounts, create the.vercel
Catalog. - Download Environment Variables: Run
vercel env pull
Download environment variables. - Installation of dependencies: Use
pnpm install
Install project dependencies. - local operation: Run
pnpm dev
Start the local development server and access thelocalhost:3000
View app.
Function Operation
- Generate Text: By calling the API of the AI SDK, you can generate a variety of text content. The sample code is as follows:
import { generateText } from 'ai-sdk';
const response = await generateText('你的提示');
console.log(response);
- Data persistence: Use Vercel Postgres to save chat logs and ensure data persistence and security. Sample code is shown below:
import { saveChat } from 'vercel-postgres';
await saveChat(userId, chatData);
- File Storage: Implement file storage through Vercel Blob, support efficient file upload and download. The sample code is as follows:
import { uploadFile } from 'vercel-blob';
const fileUrl = await uploadFile(file);
console.log(fileUrl);
- user authentication: Integrate NextAuth.js to implement user authentication and ensure application security. The sample code is as follows:
import { getSession } from 'next-auth/client';
const session = await getSession();
console.log(session);
deployments
- One-Click Deployment: Click the "One Click Deploy" button on the Vercel platform to quickly deploy your application to Vercel.
- Environment variable configuration: Configure environment variables in the Vercel dashboard to ensure that the application is running properly.
- continuous integration: With each push to a GitHub repository, Vercel automatically builds and deploys the latest version of the application.
With these steps, developers can quickly get started with AI Chatbot to build and deploy their own intelligent chatbot applications.
© Copyright notes
The article is copyrighted and should not be reproduced without permission.
Related posts
No comments...