Agno: A framework for building multimodal intelligences with memory, knowledge and tools
General Introduction
Agno is an open source Python library developed by the agno-agi team and hosted on GitHub, dedicated to making it easy for developers to build AI intelligences with memory, knowledge, and tools. It supports multimodal processing of text, images, audio, and video, and provides three core capabilities: session state storage (memory), knowledge querying (knowledge), and tool extensions (tools). agno is known for its simplicity and efficiency, and is officially said to be faster than LangGraph It is approximately 10,000 times faster with 1/50th the memory footprint and supports arbitrary language models (e.g. GPT-4o, Claude, etc.) for model-agnostic flexibility. Whether it's task automation or information processing, Agno can be implemented quickly with intuitive code. As of March 2025, Agno has received over 19,000 stars on GitHub and is very popular with developers.
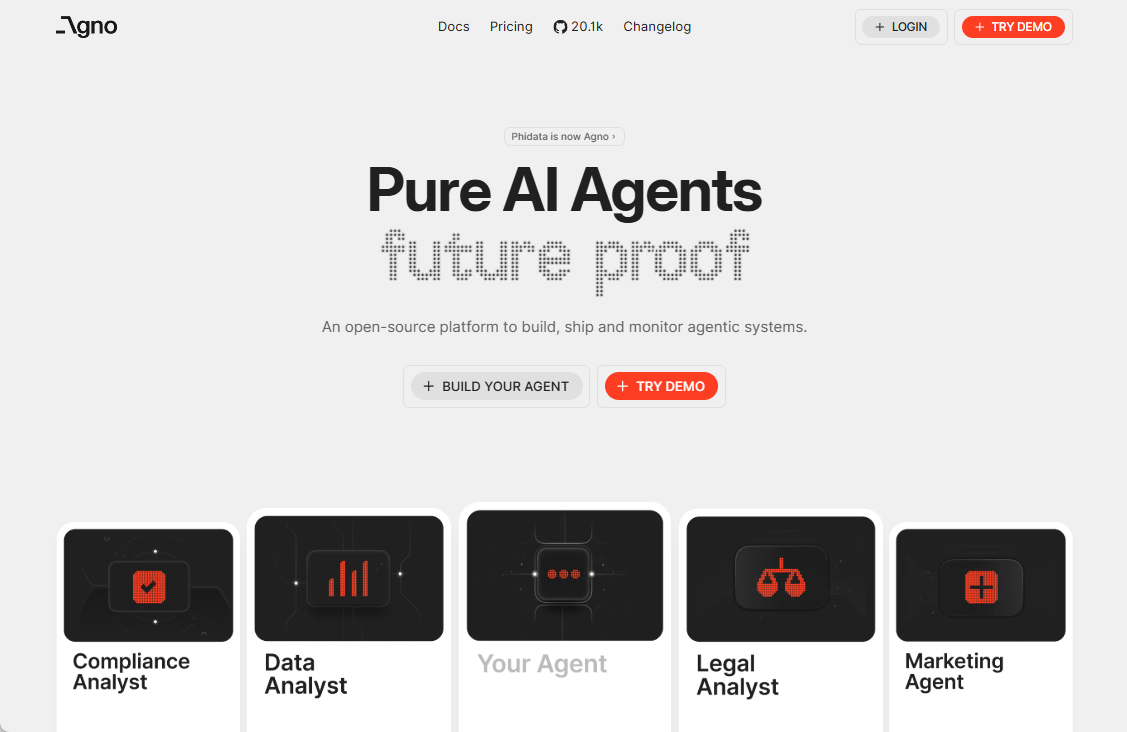
Function List
- Memory management: Stores the state of the intelligent body conversation to the database, supporting long-term contextual tracking.
- Knowledge base support: Through Agentic RAG Technical queries have a built-in knowledge base that provides accurate answers.
- tool integration: Built-in tools such as DuckDuckGo search, YFinance financial search, and support for custom extensions.
- multimodal processingSupport for text, image, audio and video input and output, applicable to a variety of scenarios.
- model independence: Compatible with any language model, no vendor restrictions and high flexibility.
- Fast Instantiation: Intelligentsia creation time as low as 2 microseconds for highly concurrent applications.
- Multi-Intelligence Collaboration: Formation of specialized intelligentsia teams to handle complex workflows.
- Structured Output: Generate formatted data such as tables to enhance the usefulness of the results.
- real time monitoring: View smartbody operational status and performance metrics via agno.com.
Using Help
Installation process
Agno is a lightweight Python framework that is easy to install and compatible with multiple operating systems. Here are the detailed steps:
1. Environmental preparation
- system requirements: Supports Windows, Linux, or macOS and requires Python 3.10 or higher.
- Check pip: Run
pip --version
Verify that pip is installed. - Cloning warehouse (optional): If you need the latest source code, run it:
git clone https://github.com/agno-agi/agno.git cd agno
2. Installation of Agno
- Installation via pip: Runs in the terminal:
pip install -U agno
- dependency: Installation on demand, e.g.
pip install openai
(OpenAI models are supported).
3. Configure the API key
Some functions require external model APIs, as in the case of OpenAI:
- Getting the key: Log in to the OpenAI official website to generate an API Key.
- Setting environment variables:
export OPENAI_API_KEY='你的密钥' # Linux/macOS set OPENAI_API_KEY=你的密钥 # Windows
4. Verification of installation
Run the following code test:
from agno.agent import Agent
from agno.models.openai import OpenAIChat
agent = Agent(model=OpenAIChat(id="gpt-4o"))
agent.print_response("Agno 有什么特点?")
If a response is returned, the installation was successful.
Main function operation flow
Using the Memory Function
Agno's Memory Management saves the session status, and the following procedure is described:
- Write code: New
agent_with_memory.py
Enter:from agno.agent import Agent from agno.models.openai import OpenAIChat agent = Agent( model=OpenAIChat(id="gpt-4o"), description="你是一个助手,能记住对话内容", markdown=True ) agent.print_response("我叫张三,你能记住吗?", stream=True) agent.print_response("我叫什么名字?", stream=True)
- (of a computer) run: In the terminal, type
python agent_with_memory.py
The intelligent experience of memorizing and responding to "Zhang San".
Using the knowledge base
Provide specialized answers via a knowledge base, e.g. load PDF:
- Installation of dependencies: Run
pip install lancedb tantivy pypdf duckduckgo-search
The - Write code: New
agent_with_knowledge.py
Enter:from agno.agent import Agent from agno.models.openai import OpenAIChat from agno.knowledge.pdf_url import PDFUrlKnowledgeBase from agno.vectordb.lancedb import LanceDb from agno.embedder.openai import OpenAIEmbedder agent = Agent( model=OpenAIChat(id="gpt-4o"), description="你是泰式美食专家!", instructions=["优先使用知识库中的泰式菜谱"], knowledge=PDFUrlKnowledgeBase( urls=["https://agno-public.s3.amazonaws.com/recipes/ThaiRecipes.pdf"], vector_db=LanceDb( uri="tmp/lancedb", table_name="recipes", embedder=OpenAIEmbedder(id="text-embedding-3-small") ) ), markdown=True ) if agent.knowledge: agent.knowledge.load() # 首次加载知识库 agent.print_response("如何制作泰式椰奶鸡汤?", stream=True)
- running result: Intelligentsia extracts recipes from PDFs to generate answers.
Use of tool extensions
Add a search tool (e.g. DuckDuckGo) to the smarts:
- Installation of dependencies: Run
pip install duckduckgo-search
The - Write code: New
agent_with_tools.py
Enter:from agno.agent import Agent from agno.models.openai import OpenAIChat from agno.tools.duckduckgo import DuckDuckGoTools agent = Agent( model=OpenAIChat(id="gpt-4o"), tools=[DuckDuckGoTools()], show_tool_calls=True, markdown=True ) agent.print_response("纽约最近发生了什么?", stream=True)
- running result: Intelligent body will call the search tool to return the latest information.
Multi-Intelligence Collaboration
Assembling teams to handle complex tasks, such as market analysis:
- Installation of dependencies: Run
pip install duckduckgo-search yfinance
The - Write code: New
agent_team.py
Enter:from agno.agent import Agent from agno.models.openai import OpenAIChat from agno.tools.duckduckgo import DuckDuckGoTools from agno.tools.yfinance import YFinanceTools web_agent = Agent( name="Web Agent", model=OpenAIChat(id="gpt-4o"), tools=[DuckDuckGoTools()], instructions=["始终提供来源"], markdown=True ) finance_agent = Agent( name="Finance Agent", model=OpenAIChat(id="gpt-4o"), tools=[YFinanceTools(stock_price=True, analyst_recommendations=True)], instructions=["用表格展示数据"], markdown=True ) team_agent = Agent( team=[web_agent, finance_agent], instructions=["协作完成任务"], markdown=True ) team_agent.print_response("AI半导体公司的市场前景如何?", stream=True)
- running result: Web Agent provides the news, Finance Agent provides the data, and collaborates to output reports.
Featured Function Operation
Combining memory, knowledge and tools
Creating an Integrated Intelligence:
- Write code: New
full_agent.py
Enter:from agno.agent import Agent from agno.models.openai import OpenAIChat from agno.knowledge.pdf_url import PDFUrlKnowledgeBase from agno.vectordb.lancedb import LanceDb from agno.embedder.openai import OpenAIEmbedder from agno.tools.duckduckgo import DuckDuckGoTools agent = Agent( model=OpenAIChat(id="gpt-4o"), description="你是美食助手,能记住用户喜好并提供菜谱", instructions=["优先使用知识库,若不足则搜索网络"], knowledge=PDFUrlKnowledgeBase( urls=["https://agno-public.s3.amazonaws.com/recipes/ThaiRecipes.pdf"], vector_db=LanceDb( uri="tmp/lancedb", table_name="recipes", embedder=OpenAIEmbedder(id="text-embedding-3-small") ) ), tools=[DuckDuckGoTools()], markdown=True ) if agent.knowledge: agent.knowledge.load() agent.print_response("我喜欢辣味,推荐一道泰式菜", stream=True) agent.print_response("我刚才说了什么喜好?", stream=True)
- running result: Intelligence will remember "like spicy flavor" and suggest related recipes.
performance testing
Verify the high efficiency of Agno:
- Running Scripts: Executed in the agno directory:
./scripts/perf_setup.sh source .venvs/perfenv/bin/activate python evals/performance/instantiation_with_tool.py
- Compare LangGraph: Run
python evals/performance/other/langgraph_instantiation.py
The results show that Agno takes about 2 microseconds to start up and has a memory footprint of about 3.75KiB.
Structured Output
Generate formatted data:
- Modify the code: operating in the financial intelligences:
finance_agent.print_response("NVDA的分析师建议是什么?", stream=True)
- running result: Returns analyst recommendations in tabular form.
By doing so, users can fully utilize Agno's memories, knowledge, and tools to build smart and efficient AI applications.
© Copyright notes
The article is copyrighted and should not be reproduced without permission.
Related posts
No comments...